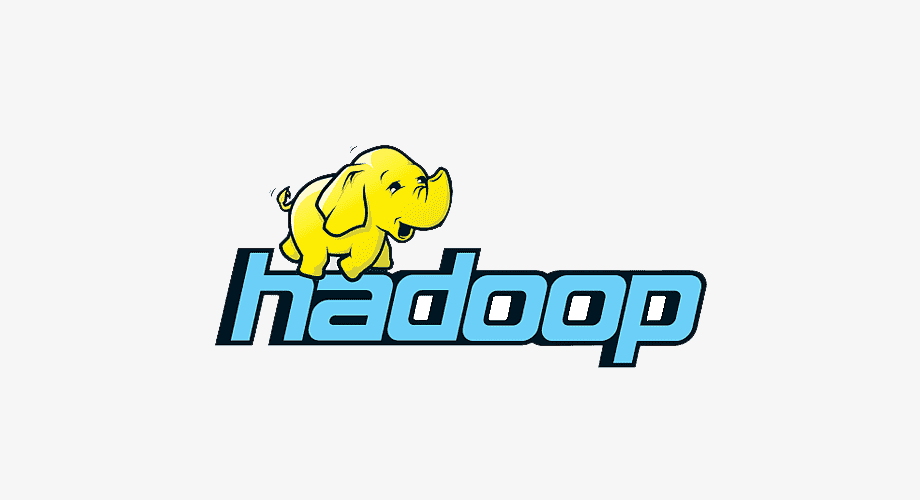
Introduction
(continuous post) The Hadoop Distributed File System (HDFS) is an indispensable component of big data ecosystems, designed to store and manage vast amounts of data across multiple nodes in a distributed fashion. WebHDFS is an HTTP REST server that provides HDFS access through a standard HTTP protocol, making it possible to interact with HDFS from various programming languages. This article aims to delve into some of the advanced concepts associated with using Python to interact with WebHDFS. We will discuss topics such as client libraries, secure communication, data ingestion, and file operations with real-world examples.
1. Overview of WebHDFS and Python
What is WebHDFS?
WebHDFS is a protocol that exposes HDFS services via RESTful APIs. It allows external systems to interact with HDFS using standard HTTP methods like GET
, PUT
, POST
, and DELETE
. WebHDFS provides a way to perform essential operations, such as reading and writing data, moving files, and managing directories, without relying on native Hadoop libraries.
Importance of Python in WebHDFS
Python plays a pivotal role in interacting with WebHDFS due to its ease of use, robustness, and versatility. In data analytics and big data domains, Python is often the language of choice due to its rich ecosystem of libraries that support data manipulation, analytics, machine learning, and more. When it comes to WebHDFS, Python libraries simplify complex operations into manageable and readable Pythonic code, thereby reducing the learning curve and development time.
Common Python Libraries for WebHDFS
Python offers various libraries to work with WebHDFS, each with its pros and cons:
hdfs
: Provides a straightforward API and supports advanced features like Kerberos authentication.pywebhdfs
: A lightweight alternative focusing solely on REST API calls.snakebite
: A pure Python client library, although it doesn’t support HTTP and is not recommended for secure HDFS installations.
Deciding on a Library
The choice of a library depends on various factors like security requirements, features needed, and ease of use. For instance, if your HDFS setup is security-intensive and uses Kerberos authentication, the hdfs
library would be a suitable choice due to its support for secure communication.
2. Setting up Python Environment
Installation of Python
Before proceeding, ensure that Python is installed on your system. For UNIX and Linux environments, Python is often pre-installed. For Windows, you can download the installer from the official Python website. It is advisable to use Python 3.x due to its updated features and broader support for libraries.
Virtual Environment
Using a virtual environment is recommended to avoid conflicts with system-wide packages. You can set up a virtual environment using venv
as follows:
python3 -m venv my_webhdfs_project
Activate the virtual environment:
- For UNIX and MacOS:
source my_webhdfs_project/bin/activate
- For Windows:
.\my_webhdfs_project\Scripts\Activate
Installing Required Libraries
Once the virtual environment is activated, you can install the necessary Python packages using pip. If you’ve decided to go with the hdfs
library, you can install it as follows:
pip install hdfs
For other libraries, the installation process is similar:
pip install pywebhdfs
or
pip install snakebite
Setting Environment Variables
Depending on your setup, you might need to set environment variables like HADOOP_CONF_DIR
to point to the directory containing Hadoop’s configuration files (core-site.xml
and hdfs-site.xml
).
These expanded sections should provide a more comprehensive understanding of the role of Python in WebHDFS and how to set up the Python environment for WebHDFS interactions. The choice of library and the proper setup of the Python environment are foundational steps for advanced operations, which will be discussed in subsequent sections.
3. Advanced File Operations
Upload
To upload large files, you can use the write()
method with the buffersize
parameter to define the chunk size.
from hdfs import InsecureClient
client = InsecureClient('http://localhost:50070')
with open('large_file.txt', 'rb') as file:
client.write('/user/data/large_file.txt', file, buffersize=65536)
Download
Similarly, when downloading, you can specify a buffersize
to control data flow.
client.read('/user/data/large_file.txt', buffersize=65536)
Append
Appending data to an existing file can be done via the write()
method by setting the overwrite
parameter to False
.
client.write('/user/data/existing_file.txt', data, overwrite=False)
4. Data Serialization and Deserialization
While dealing with complex data types like dictionaries or arrays, consider using JSON or Avro for serialization and deserialization.
5. Security Considerations
Kerberos Authentication
hdfs
library supports Kerberos authentication, which can be configured using the mutual_auth
parameter.
HTTPS Support
For secure communication, HTTPS can be enabled. However, you need to ensure that your Hadoop configuration files reflect this change.
6. Performance Optimization
Performance optimization is a critical aspect of any big data operation, and interactions with WebHDFS are no exception. WebHDFS, though convenient, often adds an overhead due to its RESTful nature. Therefore, it becomes paramount to leverage various strategies to enhance the performance of data operations. In this expanded section, we’ll discuss several facets, including DataNode locality, connection pooling, and parallelism, which can significantly improve the performance of your Python-based WebHDFS operations.
Understanding DataNode Locality
In a distributed file system like HDFS, data is stored across multiple nodes. DataNode locality refers to the strategy where computing tasks are scheduled to run on the same node where the data resides. This approach minimizes network overhead and speeds up data processing.
While WebHDFS does not automatically leverage DataNode locality, it’s possible to implement this manually. For example, you can query the DataNode locations for specific blocks of a file using the GET_BLOCK_LOCATIONS
operation and then target your read or write operations to use the local DataNode.
Connection Pooling
HTTP connections to WebHDFS can be resource-intensive. Each RESTful API call involves setting up a new connection, SSL/TLS handshakes if security is enabled, and finally, tearing down the connection. Connection pooling allows you to reuse existing connections, reducing the overhead of these operations.
Libraries like urllib3
offer connection pooling features, and they can be integrated into your Python WebHDFS client to optimize performance. Connection pooling is particularly beneficial in scenarios involving multiple small read or write operations.
Parallelism
For large files or massive datasets, you may benefit from parallel read and write operations. This involves breaking the file into smaller chunks and processing these chunks simultaneously, either within the same machine using multi-threading or across different machines in a distributed manner.
Python’s concurrent.futures
library can be used to implement simple multi-threading. For more complex scenarios involving multiple machines, you might consider using a distributed computing framework like Apache Spark, which has built-in support for HDFS and can be easily interfaced with Python.
Data Compression
Data compression algorithms like Gzip or Snappy can be used to reduce the volume of data transmitted over the network. This is particularly useful for write-heavy workloads or when the network is a bottleneck. Most HDFS clients offer some form of support for compressed data, and this can be exploited to gain performance benefits.
Batch Operations
Whenever possible, batch multiple operations together to reduce the number of API calls. For instance, if you need to create multiple directories or upload several files, try to batch these operations into a single API call. This is often more efficient than issuing separate calls for each operation.
Monitoring and Profiling
Finally, no performance optimization strategy is complete without proper monitoring and profiling. Tools like Apache Ambari or custom logging can help you identify bottlenecks in your WebHDFS operations. Use this data to tweak your strategies further and continuously optimize performance.
By adopting these performance optimization techniques, you can significantly enhance the efficiency of your Python-based WebHDFS operations. These strategies range from simple changes in how you manage HTTP connections to more complex approaches involving DataNode locality and parallel processing. Each method has its own set of advantages and trade-offs, so it’s important to analyze your specific use case to determine which techniques will offer the most benefit.
7. Real-world Use Case: Data Ingestion Pipeline
In big data scenarios, data ingestion pipelines serve as the foundation for moving data from various sources into a centralized storage system like HDFS. With an ever-growing need for businesses to analyze disparate forms of data, ranging from logs and streams to databases and user-generated content, a data ingestion pipeline acts as the first layer of a data-intensive application. In this expanded section, we will explore how Python and WebHDFS can be employed to build a robust and efficient data ingestion pipeline.
The Architecture of a Data Ingestion Pipeline
A typical data ingestion pipeline consists of three main components:
- Data Sources: These can be varied, including databases, log files, real-time streams, or APIs.
- Ingestion Layer: This is the layer where the data is processed, transformed, and loaded into HDFS.
- Data Storage: Usually, this is a distributed storage system like HDFS for big data applications.
Python’s versatility allows you to interact seamlessly with a plethora of data sources, while WebHDFS provides a convenient way to ingest this data into HDFS.
Data Source Integration
Python libraries can connect to various types of data sources:
- Databases like MySQL, PostgreSQL can be accessed using libraries such as
psycopg2
orMySQLdb
. - Log files can be read natively or via log collectors like Fluentd.
- Real-time streams like Kafka can be interfaced using the
confluent-kafka
Python package.
For example, you could read data from a PostgreSQL database as follows:
import psycopg2
conn = psycopg2.connect(database="mydatabase", user="user", password="password", host="127.0.0.1", port="5432")
cursor = conn.cursor()
cursor.execute("SELECT * FROM table_name;")
data = cursor.fetchall()
Data Preprocessing and Transformation
Before the data is ingested into HDFS, it often needs to be cleaned, transformed, or enriched. Python’s pandas
library is particularly useful for these tasks.
import pandas as pd
df = pd.DataFrame(data, columns=['column1', 'column2'])
df['new_column'] = df['column1'] + df['column2']
Data Ingestion into HDFS
Once the data is prepared, it can be ingested into HDFS. Here is where WebHDFS comes into play:
from hdfs import InsecureClient
client = InsecureClient('http://localhost:50070')
with client.write('/user/data/ingested_data.csv') as writer:
df.to_csv(writer)
Scheduling and Automation
In real-world scenarios, data ingestion is a continuous process that needs to be automated. Python scripts handling data ingestion can be scheduled to run at specific intervals using task schedulers like Cron in UNIX systems or Task Scheduler in Windows. For more complex workflows, orchestration tools like Apache Airflow can be used.
Monitoring and Logging
It’s crucial to monitor the data ingestion pipeline for any failures or performance bottlenecks. Python’s logging library can be integrated into your script for logging purposes, and monitoring solutions like Prometheus can be employed to keep track of the pipeline’s health.
By strategically employing Python and WebHDFS, you can create a robust, scalable, and efficient data ingestion pipeline. Python’s extensive ecosystem provides the tools for source integration, data transformation, and automation, while WebHDFS ensures that the data lands safely in your HDFS cluster. With performance optimizations and monitoring in place, such a pipeline becomes an invaluable asset in your data engineering toolkit.
8. Conclusion
Python-based WebHDFS interactions offer a plethora of possibilities. Not only does it serve as a medium to perform basic file operations, but it also opens doors to advanced operations like secure communication, performance optimization, and complex data handling.
By mastering these advanced concepts, you position yourself to leverage the full capabilities of HDFS, thereby optimizing your big data solutions for both functionality and performance.